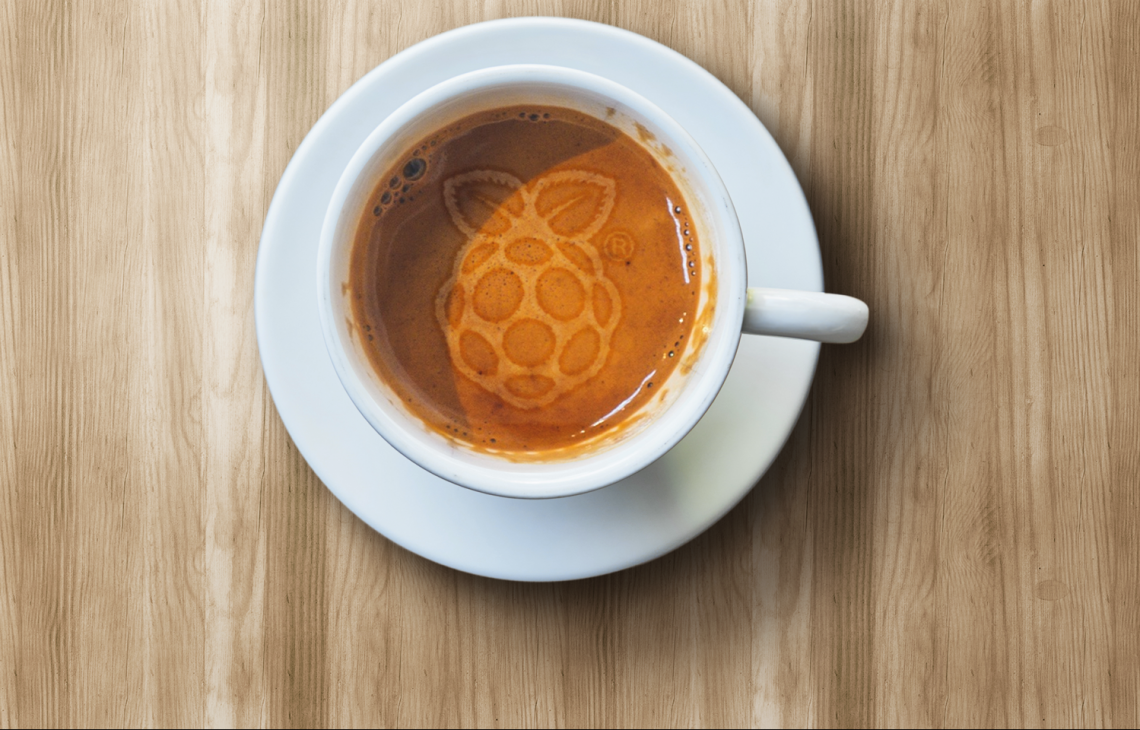
Tall Frappe WiPi Latte Part II – Software
WiFi connected smart coffee machine #thedream.
I have a Delonghi Magnifica – a magnificent coffee generating machine with just flaw. You have to physically press buttons and turn dials. So we better do something about that!
The Plan:
Add a WiFi connected raspberry pi and touchscreen. Create a web interface for the raspberry pi so we can control it from any device. Create a library of drinks so the settings can be changed automatically.
Some things to note:
- All python files must be executable “$ chmod +x myscript.py”. Some people may be tempted to go down the modifying the sudoers file to give root permission to everything. Don’t do this unless you want the Chinese government’s Starbucks devision monitoring your coffee habits!
Libraries
It’s worth noting at this point we will be using python 3. Therefore it’s really important that you use pip3 otherwise we won’t be able to access the libraries!
Pre-requisites
We’re going to be building this headless. That means that’ll we’ll be remotely building the system from another computer instead of directly on the raspberry pi. I’m going to Assume some knowledge of how to use ssh, ftp + some basic command line commands but none of it should be too complicated and I’ll include every step so at best it’ll be copy and paste, at worst they’ll be a small amount of googling involved!
First step is to get ourself a fresh copy of raspbian. I’m going to be using stretch but most versions should work. So to start off lets flash raspbian to a micro SD. We’ll then remove the SD and put it back into the computer as we need to modify a few settings in the boot directory.
We need to add two files. One name “ssh” with nothing in it and no file extension. This will enable ssh once the pi is fired up for the first time. And second a file called wpa_supplicant.config we’ll add our wifi details in here as per the raspberry pi documentation.
Next I found to use my small get touchscreen working I had to modify a few settings in config.txt.
- overscan = 1
- hdmi_group =1
- hdmi_mode = 2
Sweet! let’s slide the SD card in the pi and fire it up!
Setup
Once it’s loaded we can SSH into the pi without needing to know the ip by the command line code
ssh pi@raspberrypi.local
Then follow the onscreen instructions and enter the default password “raspberry”.
Awesome we’re in! Next we’re going to modify a few settings so type:
sudo raspi-config
This will bring up the pi’s configuration menu (effectively it’s bios). We’ll start by using the arrow and enter keys to change the password. Go to option 1 “Change User Password” and follow the instructions. to advanced options (7) and overscan (A2). For my touchscreen i needed to disable overscan compensation to get the display to fill the screen. Next go back to advanced options and chose Expand Filesystem (A1). This will ensure the SD card’s storage is all full available. And finally go to Network Options (2) and Hostname (N1). This is where we’ll set the URL we’ll use to ssh into the pi and access our web app. I set mine as coffee. Finally hit <Finish> and reboot your pi. This will disconnect your terminal window and restart the pi.
Now we’ll need to use a different command to login to the pi this time as we’ve changed the hostname. For me this was
ssh pi@coffee.local
Great! Now we can start downloading all the libraries we’re gonna need.
First we’ll have to make sure the library manager in the pi is up to date or it’ll throw a hissy fit when we try the next step. Type
sudo apt-get update && sudo apt-get upgrade
We’re going to use pip to install our packages. This should already be pre-installed on raspbian but you can check by typing
pip3 --version
If it spits out the version number we’re all good! If it doesn’t do a google and you’ll find out how to fix it no problem!
Now install Flask by typing.
pip install -U Flask
The -U command performs any updates we might need at the same time. Finally install rpi-gpio. Again it should already be installed but it doesn’t hurt to check.
sudo apt-get install rpi.gpio
And that is our pi all setup! Now we’re going to start by getting our flask server up and running. Start by creating a new file by typing the following. You’ll need to press enter after typing each line.
mkdir kiosk
cd kiosk
mkdir webapp
cd webapp
sudo nano app.py
You should now be in the nano text editor. This is a command line text editor built into terminal. When we start building the app proper we’ll use an ftp client but we just want to check everything is setup correctly first.
Paste the following code into the file the press ctrl + x and then y and enter.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, World!"
Now open up a web browser and visit www.coffee.local (if you’re on a windows machine you’ll need to install bonjour. you can get the working via the apple print services found here.
If all goes to plan you should see Hello, World! in your browser window. Nice.
Coffee Machine Interface
hi
Web App
Now we’re going to switch to FTP for the rest as it’s much more efficient for building apps. I usually use FileZilla as it’s free and works ok but you’re welcome to use any FTP client you like! So login into the pi’s file system with the url coffee.local the username pi and password raspberry. Next navigate to the folder kiosk>>webapp and copy the files attached below to this directory. You’ll need to overwrite the app.py file we’ve already created.
I’m not going to go through everyline of code but I’ll explain the basic functionality
Alexa Integration
hi
Touch Screen
hi
And Done
You should now have a working wifi Alexa connected coffee machine!
https://projects.raspberrypi.org/en/projects/python-web-server-with-flask/3
https://obrienlabs.net/setup-kiosk-ubuntu-chromium/
https://newscrewdriver.com/2018/02/07/launching-a-flask-web-app-on-startup/
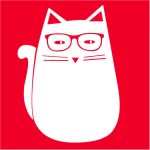
Ollie
An electromechanical systems engineer with passion for all things technology, design and feline.
You May Also Like
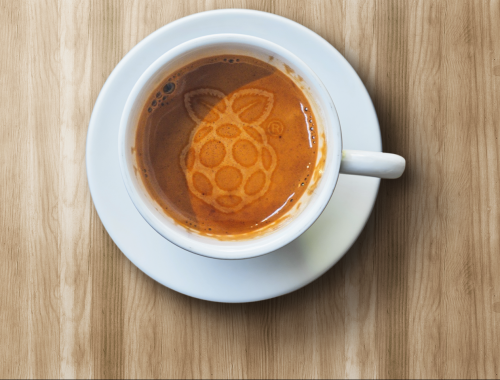
Tall Frappe WiPi Latte Part I – Hacking In
4th December 2019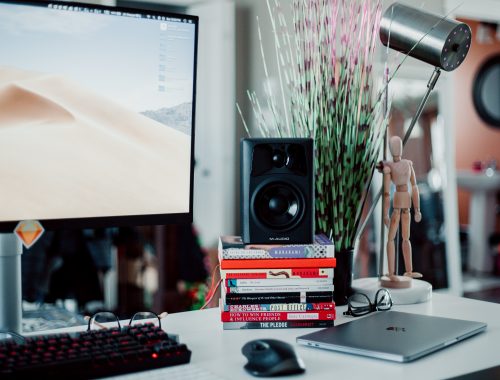